In azure vnets allow you to separate out parts of your cloud infrastructure into different network segments. This structure is almost the equivalent of building or setting up a new office location except the cloud has made it a lot faster. Although creating vnet’s in azure can be completed really easy, automating this deployment can make sure that there is a consistent deployment setup across networks.
To create a vnet in azure using terraform, we will be building the following resources in terraform:
- An Azure resource group
- Then a virtual ntework
- Allocate a CIDR range for the virtual network
- A default subnet
Building your resource group in terraform
The resource group is what holds all the resources that share a common lifecycle together. This makes it easier to view everything in a logical unit. Create a new folder in the directory of your choice and create a file called spoke-network.tf. Inside the file place the following code in their.
terraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "=3.0.0"
}
}
}
# Configure the Microsoft Azure Provider
provider "azurerm" {
features {}
}
#build azure resource group
resource "azurerm_resource_group" "main" {
name = "mainnetwork"
location = "eastus"
}
Here we declared our required providers for this code. The resource group is being deployed in the “eastus” region and called “mainnetwork”
Create a vnet in azure
The vnet is dependent on the resource group being available. Now lets add the resource block for the virtual network below our resource group.
Resource "azurerm_virtual_network" "mainnetwork" {
name = "mainnetwork"
location = azurerm_resource_group.main.location
resource_group_name = azurerm_resource_group.main.name
address_space = ["10.0.0.0/16"]
}
This deploys a virtual network called “mainnetwork” inside the “mainnetwork” resource group with an address space of 10.0.0.0/8. This will then allow you to deploy multiple subnets in that range. There are additional options that can be declared in the virtual network resource block such as:
- ddos_protection_plan
- dns_servers
- edge_zone
Building your subnets in separate terraform blocks
We could add our subnets to the current azurerm_virtual_network block, but separating them out makes it easier when needing to reference them for other things if the terraform project grew.
Resource "azurerm_subnet" "devsubnet" {
name = "dev-subnet"
resource_group_name = azurerm_resource_group.main.name
address_prefixes = ["10.0.1.0/24"]
virtual_network_name = azurerm_virtual_network.mainnetwork.name
}
Resource "azurerm_subnet" "testsubnet" {
name = "test-subnet"
resource_group_name = azurerm_resource_group.main.name
address_prefixes = ["10.0.2.0/24"]
virtual_network_name = azurerm_virtual_network.mainnetwork.name
}
To create the completion of the vnet in azure using terraform we built 2 subnets. The dev and test subnet will both be linked to the “mainnetwork” virtual network. When finished we will have a simple vnet with 2 subnets pictured below
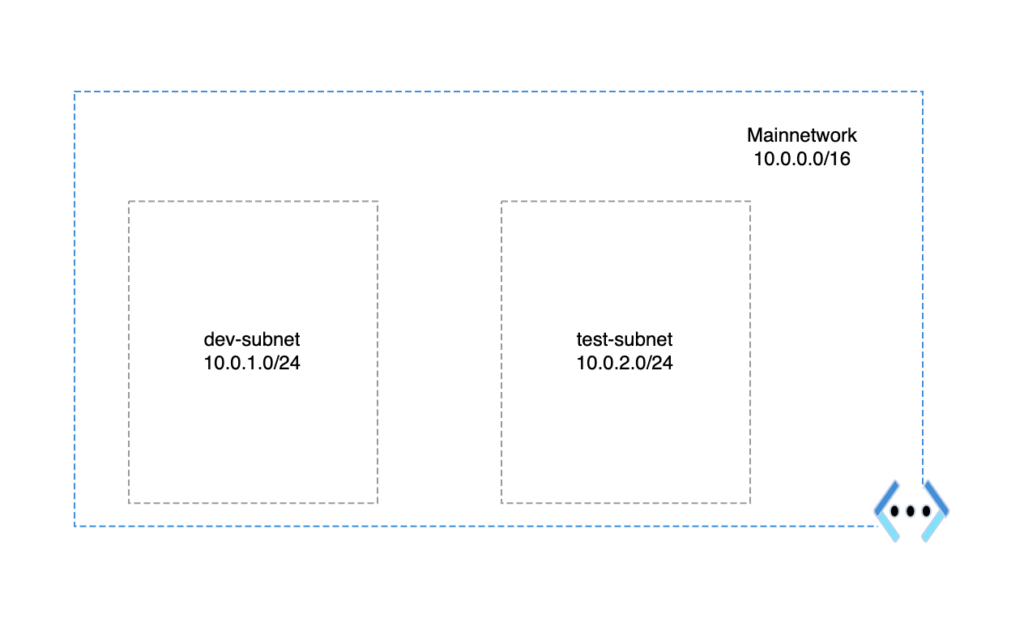
Conclusion
Once finished the final deployment of terraform code should not take too long. The great thing is this template will be a great starting point to continue with building out an entire azure network from scratch and peering along with other virtual networks.
terraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "=3.0.0"
}
}
}
# Configure the Microsoft Azure Provider
provider "azurerm" {
features {}
}
#build azure resource group
resource "azurerm_resource_group" "main" {
name = "mainnetwork"
location = "eastus"
}
Resource "azurerm_virtual_network" "mainnetwork" {
name = "mainnetwork"
location = azurerm_resource_group.main.location
resource_group_name = azurerm_resource_group.main.name
address_space = ["10.0.0.0/16"]
}
#build devsubnet and link to mainnetwork virtual network
Resource "azurerm_subnet" "devsubnet" {
name = "dev-subnet"
resource_group_name = azurerm_resource_group.main.name
address_prefixes = ["10.0.1.0/24"]
virtual_network_name = azurerm_virtual_network.mainnetwork.name
}
#build testsubnet and link to mainnetwork virtual network
Resource "azurerm_subnet" "testsubnet" {
name = "test-subnet"
resource_group_name = azurerm_resource_group.main.name
address_prefixes = ["10.0.2.0/24"]
virtual_network_name = azurerm_virtual_network.mainnetwork.name
}
Good introduction to building infrastructure in Azure with Terraform