We looked at how to create and destroy a straightforward EC2 instance in the last post on Terraform. This guide will walk you through how to create an aws key pair using terraform. Watch this video below if you would like to see it step by step in action. Code snippets below as well.
How to create an ssh key using windows
Modern cloud services and other computer-dependent services are made practical and cost-effective by automation, which is made possible by SSH keys. When managed correctly, they provide convenience and enhanced security. To create an aws key pair using terraform:
- Open the command prompt and type in “ssh-keygen -t rsa -b 2048”
- Choose the location that you want your key to be saved along with a key name
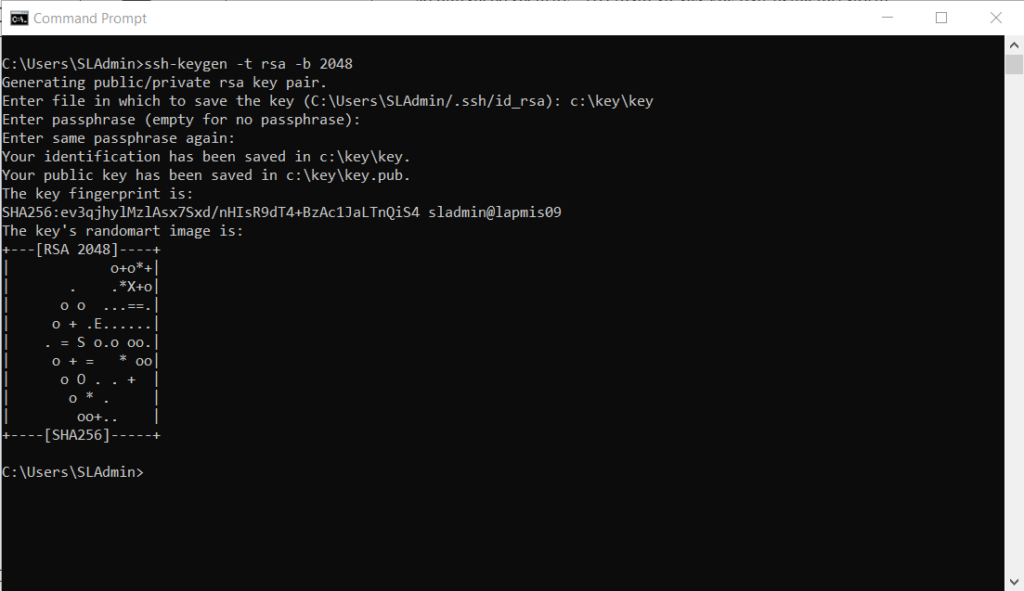
ssh-keygen command
How to import the ssh key into terraform
Now that we have our public and private key saved lets create our aws key pair using terraform. If you dont have a template to go off of, you can utilize the one below. You will need to setup your own terraform cloud account.
terraform {
cloud {
organization = "<YOUR TERRAFORM CLOUD ORGANIZATION NAME>"
workspaces {
name = "<YOUR WORKSPACE NAME>"
}
}
required_providers {
aws = {
source = "hashicorp/aws"
version = "= 4.27.0"
}
}
}
provider "aws" {
region = "us-east-2"
}
#EC2 instance details
resource "aws_instance" "instance1" {
ami = "ami-0568773882d492fc8"
instance_type = "t2.micro"
vpc_security_group_ids = [aws_security_group.main.id]
key_name = "aws_key"
tags = {
Name = "linux"
}
depends_on = [
aws_key_pair.devkey
]
}
resource "aws_security_group" "main" {
egress = [
{
cidr_blocks = [ "0.0.0.0/0", ]
description = ""
from_port = 0
ipv6_cidr_blocks = []
prefix_list_ids = []
protocol = "-1"
security_groups = []
self = false
to_port = 0
}
]
ingress = [
{
cidr_blocks = [ "0.0.0.0/0", ]
description = ""
from_port = 22
ipv6_cidr_blocks = []
prefix_list_ids = []
protocol = "tcp"
security_groups = []
self = false
to_port = 22
}
]
}
resource "aws_key_pair" "devkey" {
key_name = "aws_key"
public_key = "PASTE YOUR SAVED .PUB KEY HERE"
}
then under the resource “aws_instance” block, you need to make sure that the ami matches with the region that you choose. Open the .pub key file in the location you saved it. Copy the key and place it in the “public_key” section of the terraform code.
Now if you look above in the “aws_instance” section you will see that the block has a depends_on section. This is needed because the aws_key_pair needs to be created before the instance gets created since it relies on the key. Without that the terraform will fail. Once you have the code all filled out, you can then run:
- First Terraform init
- Then Terraform Plan to make sure its all correct
- Finally Terraform Apply
Now this will create this key and resource in the default vpc for just testing purposes.

Connect to EC2 Instance
Download putty here and we will be using the tool puttgen, putty and pageant. Once installed open puttygen and import your private key file.
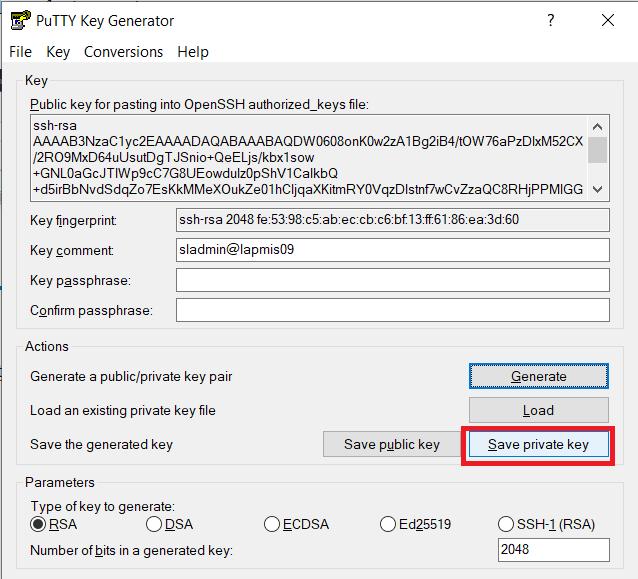
Save the key without a passphrase. Then Save that key in the ppk format. You will then need to run the pageant application, Right click the icon in the bottom right hand corner and click add key. Choose your .ppk key.
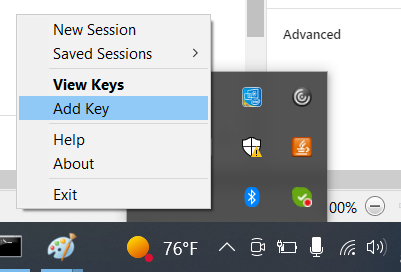
Once the key is added you can then go to your ec2 instance in amazon and get the public dns name of your instance.
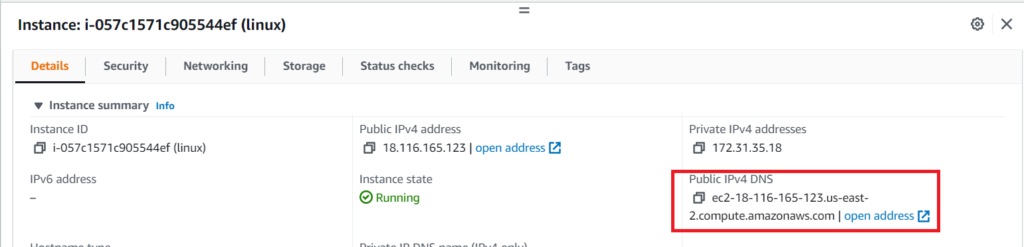
ssh into your instance with that dns name and the username can be found by selecting the ec2 instance and going to connect. That screen will show you the user to login with.
Conclusion
So this lab showed you how to create an aws key pair using terraform. As you to build more environments this simple process can not only help you be more secure in how you connect to your host but also help you learn about how aws key pairs work. This can also be done by creating the key pair in aws first and importing it in.